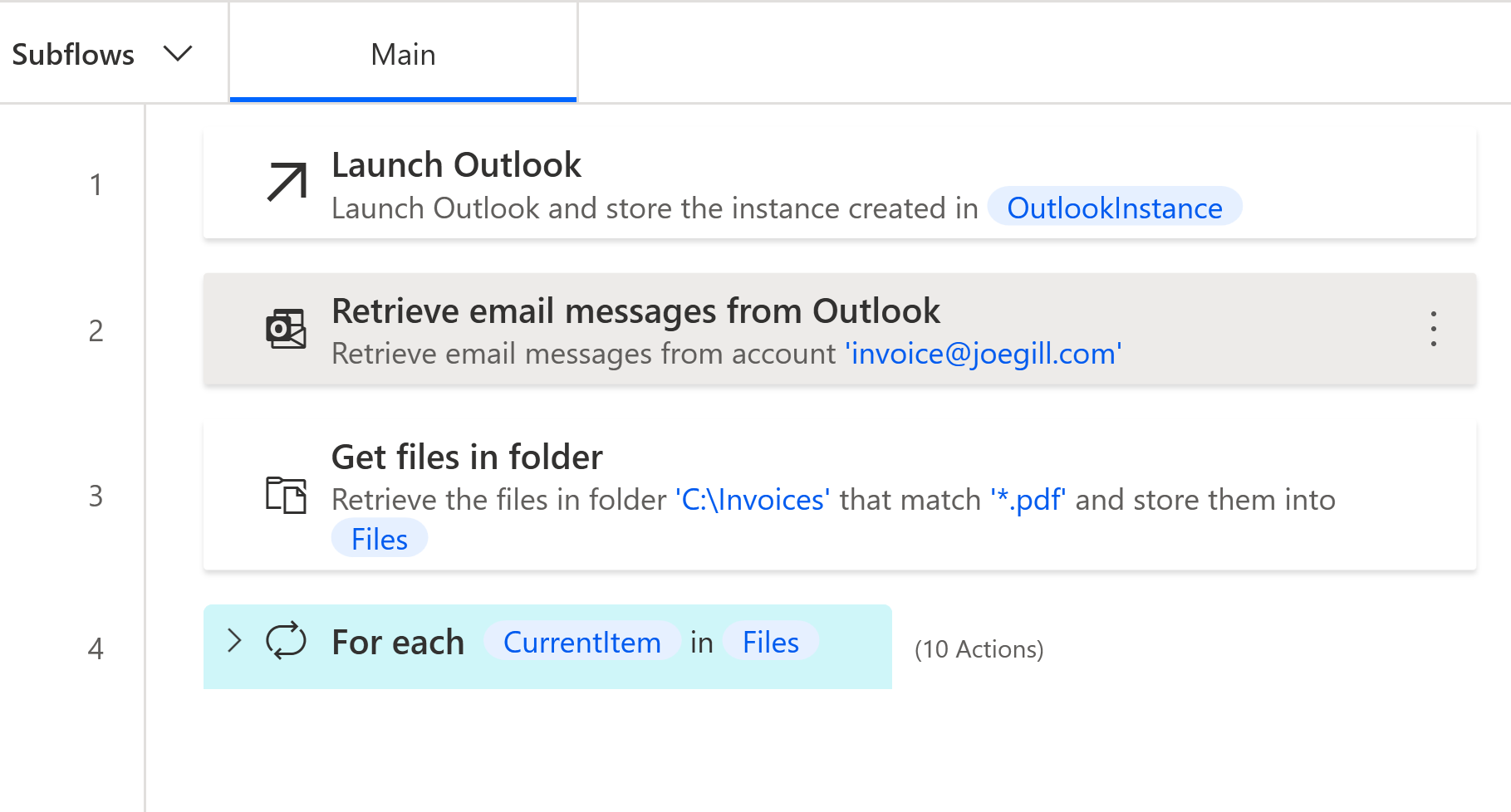
Playwright is an open-source web testing library developed by Microsoft that can be used for testing modern web apps. You can use Playwright to create test scripts for your Power Apps and use these to run automated tests.
Some of the benefits of using Playwright are
Here is an example in Node that starts an instance of the Chromium browser, waits for a web page to load then saves a screen shot of the page.
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://www.joegill.com');
await page.screenshot({path: 'joegill.com.png'});
await browser.close();
})();
Full details on Playwright and how to install it for your given environment can be found here To install Playwright for Node run the following
npm i -D @playwright/test
Playwright comes with utilities to record your browser actions to generate test scripts. You do this by running the Node executable playwright command with the codegen argument. Adding the URL of my model-driven Power App as a parameter will open a browser session where the user’s actions are recorded in the Playwright Inspector.
npx playwright codegen https://joegill.crm4.dynamics.com/main.aspx?appid=0bb0%15c-457b-eb11-a832-0022489adc1
Any actions I carry out are added to the recorded steps. Here I have created a new account record with its industry set to Consulting
Once I finished recording I can select the language for my test script and simply copy it into my code editor.
One of the major benefits of Playwright is that it can auto-wait for checks to be completed before executing an action. The range of checks includes auto waiting for
Xrm.Utility
You can use the Model-Driven app client-side Javascript library from your Playwright scripts. Here is a snippet that saves a new record and then wait for the entityId to be populated before continuing. It then gets the user name and entity id and saves a screenshot with those details
await page.click('[aria-label="Save"]');
await page.waitForFunction('Xrm.Utility.getPageContext().input.entityId !== null');
const account = await page.evaluate(() => {
return {
username: Xrm.Utility.getGlobalContext().userSettings.userName,
id: Xrm.Utility.getPageContext().input.entityId
}
});
await page.screenshot({ path: account.username + account.id + '.png', fullPage: true });
If you use the CodeGen options to record your steps then it will record your user name and password in the script. You need to create parameters for these.
You should also create a parameter for your URL and Appid so you can run the same tests in different environments.
Using a tool like Playwright to create automated tests will increase the quality and coverage of your testing. Automated tests are cost effective as tests can be run repeatablably without the need for human intervention. Recording screenshots or video as part of the tests is allows the test results to be viewed and checked.
It is worth checking out if you are interested in the automated testing of your Power Apps.